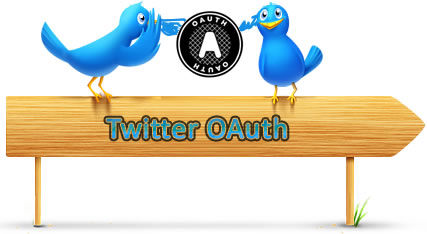
Using the new PHP-Twitter API OAuth class
Since August 16 when TwitterAPI team announced the shutting down of basic authentication of Twitter API, which was deprecated on August 31, 2010, all client and browser based tweet application have been on the run for update. I think that’s the currently web buzz on tweet plugins/modules.
The fact is, Twitter API now accepts communication via OAuth and OAuth only. This gives me a big hit on all my blogs and codes …..UPDATE!
Prior to this OAuth enforcement agent, I use a nice script by Tijs Verkoyen and it works pretty good. He posted an update for the new Twitter OAuth but it’ seems there are lots of headache on it — from users comment.
I gave it a shot and this is how I got it working after some tries. You can grab the new OAuth powered Twitter API class by Tijs Verkoyen here
This is a quick example on how to use the class:
<?php // require require_once 'twitter.php'; // create instance $twitter = new Twitter('<your-consumer-key>', '<your-consumer-secret>'); // get a request token $twitter->oAuthRequestToken('<your-callback-url>'); // authorize if(!isset($_GET['oauth_token'])) $twitter->oAuthAuthorize(); // get tokens $response = $twitter->oAuthAccessToken($_GET['oauth_token'], $_GET['oauth_verifier']); // output, you can use the token for setOAuthToken and setOAuthTokenSecret var_dump($response); ?>
At this point, you need to create a consumer key and a consumer secret. Consumer? All I want to do is tweet, who is the consumer and what is been consumed? Please ask Twitter by creating an application for your blog or project.
Firstly, go to the Twitter API site to create the necessary keys. Note that you need to log in before you can continue. Fill in the necessary fields like
‘Application Name‘ – this is the name that will appear on your tweet source as in ‘about 1 hour ago via RWB’
‘Description‘ – The description if available.
‘Application Website‘
‘Application Type‘ – Browser for web applications.
‘Callback URL‘ – The return URL after authentication.(You really don’t need this for this class)
‘Access type‘ – Make sure you give your app READ AND WRITE access
Once you are done filling out the form and it submitted, you will be redirected to the APP profile page where the consumer key and consumer secret is residing.
Copy these consumer key and secret; you will also need to copy the ‘Access Token‘. You can find this by clicking on the ‘My Access Token‘ link on the right corner of the page. Copy the Access Token (oauth_token) and the Access Token Secret (oauth_token_secret). Back to the class instance; paste each string/key in the appropriate places.
// create instance $twitter = new Twitter('[your-consumer-key]', '[your-consumer-secret]');
Referring to the code I updated to for most of my APP tweets:
public function post_twitter($text, $url, $return=0){ require_once('twitter.php'); $twitter = new Twitter('consumer-key', 'consumer-secret'); //set Access Token $twitter->setOAuthToken('your-OAuthToken'); //set Access Token Secret $twitter->setOAuthTokenSecret('your-OAuthSecret'); //This is just for bit.ly $bitly = self::make_bitly($url,'json'); $text_status = substr($text,0,128).'... '.$bitly; //Update the status $response = $twitter->statusesUpdate($text_status); if($return == 1): return $text_status; endif; //var_dump($response); //Note: this is the response I got when the status has already been posted //Fatal error: Uncaught exception 'TwitterException' with message 'Status is a duplicate.' //die(); }
With this short lines of code, I found this pretty easy to use and I was able to call my APP twittering back to life.
I think is time for you to update too.
Please drop a comment, ask any question or share your preferred knowledge about this topic.
Thanks for reading.
Hi,you could put a screenshot of the following text:
"Copy these consumer key and secret; you will also need to copy the ‘Access Token‘. You can find this by clicking on the ‘My Access Token‘ link on the right corner of the page. Copy the Access Token (oauth_token) and the Access Token Secret (oauth_token_secret). Back to the class instance; paste each string/key in the appropriate places."
Thanks
Thanks Daniel.
Thank!
You are welcome
Hello, I have another question .. what if I get the tokens
(oauth_token and oauth_token_secret) from the $ response = $ twitter-
> OAuthAccessToken (), like I can save to a text file?
Regards
If you notice Daniel, I didn't bother to make use of the callback URL or request for access token. You can always set your access token you copied from 'My Access Token' page.
/set Access Token
$twitter->setOAuthToken('your-OAuthToken');
//set Access Token Secret
$twitter->setOAuthTokenSecret('your-OAuthSecret');
I will advise you to make use of the above lines of code instead of
// get a request token
$twitter->oAuthRequestToken('<your-callback-url>');
// authorize
if(!isset($_GET['oauth_token'])) $twitter->oAuthAuthorize();
// get tokens
$response = $twitter->oAuthAccessToken($_GET['oauth_token'], $_GET['oauth_verifier']);
ok, thanks a lot 🙂
You are most welcome Daniel.
This one actually really works! Love you!
Glad it worked for you Chris.
Great work!
Finally, finally something that works without spending hours of trying! After carefully looking how you posted a tweet, it was easy to make tweets go away or show them all on a page.
Thanks a lot.
You are welcome Peter.
Can you confirm where these two pieces of code go. Are they separate class pages, or is any of it added to "Twitter.php. I can't get it to work. Am I altering Twitter Class page at all?
Thanks!
@John
You don't need to alter the class file(twitter.php); all you need to do is include it, create an object from it and work with the object of that class as in
require_once('twitter.php'); //include it
$twitter = new Twitter('consumer-key', 'consumer-secret'); //create an object of the class
//then work with the object
$twitter->setOAuthToken('your-OAuthToken');
$twitter->setOAuthTokenSecret('your-OAuthSecret');
$response = $twitter->statusesUpdate($text_status);
So this code is meant to be on a separate file. I hope you get that right.
Worked first time!
(maniacal laughter)
Thanks to you and to Tijs Verkoyen.
Hey, I can't find button "my access token" on right.
In fact the page looks a bit different. I mean I have buttons on top, but not on the right.
Where else I can get permanent my token and Secret?
Check it properly, it right there under 'Edit Application Settings', 'Manage Domains' and 'View your applications'
Thanks for the writeup. It makes much more sense than anything else I found about this API. Sadly, it doesn't work. ;_(
Warning: curl_setopt_array() [function.curl-setopt-array]: CURLOPT_FOLLOWLOCATION cannot be activated when in safe_mode or an open_basedir is set in /www/htdocs/w008d927/www2/twitter.php on line 446
{"request":"/1/statuses/update.json","error":"Could not authenticate you."}
What now?!
I guess u are the first to say this is not working. Perhaps u need to check/correct the cause of that warning or check ur code.
Me too..double check the credentials and all okay..always got this result:
{"request":"/1/statuses/update.json","error":"Could not authenticate you."}
@Fahmi,
Perhaps there is something wrong in the keys; this always occur with cut and paste a lot. This script is still working for me as I type. It works fine on my APPs. Can u post ur declaration here for us to see?
Hi Silas,
Everything is working perfect this way, but I would like to use this library login in with my twitter username and password. Is it possible?
Thanks.
At the beginning of my post, I said:
“The fact is, Twitter API now accepts communication via OAuth and OAuth only.”
Are you able to provide sample code that utilizes the callback URL and request for the access token? Rather than the hard coded oauth_token and oauth_verifier? thank you!!
Ah sorry about last post. I was incorrectly using as https://twitter.com/oauth/request_token
It should be your own website address!
Thanks Silas! How to update a status from a PHP script running as a CRON job had been frustrating me for days. Thanks!
You are most welcome Andy. cool it worked for you. thanks to Tijs.
Thanks Silas!
It seems twitter changed the page and I couldn’t find the page for the token key and secret any more. Also “my token access” button. Any updates on this matter?
There is no change on Twitter’s page. All links’ position still remain the same. Are you sure you are access right URL? http://dev.twitter.com/apps/new
Thank you Silas for this excellent piece of work. In first instance I had the error “Timestamp out of bound”, because the server time was not right. Yes takes a lot of time to find that out such a small error …
You are welcome.
Thanks for your code, it is pretty fit to my application, Can I post msg to some other users ?
If you mean tweet @A-User, then yes. if otherwise, I don’t think so.
it’s nice to share this information. looking forward to reading more.