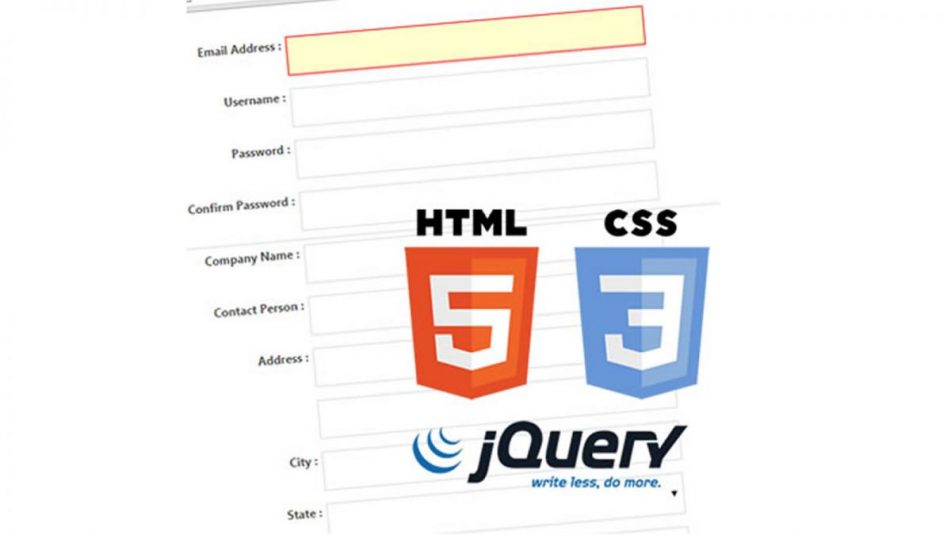
HowTo: Quick form validation with jQuery
Have you ever thought of a better way to validate each input field of a long form without a sequence validation? Well, I was forced to have my way around it and I will be sharing a simple method using jQuery.
The truth about form is that:
- you are not sure if your users will enter the appropriate details you are requesting for;
- so you need to validate the user’s entry before submitting it to the server.
But, remember that javascript validation should not be the only medium for your validation; it’s useful when you don’t want your user to revisit the form page for some minor error correction. So, I will suggest taking it as a good practice to validate on the client-side before submitting to the server.
It’s so funny sometimes when people omit or enter invalid information whilst filling a form; “you need to correct the old man, he’s probably losing his sight” – *whispering.
So, let’s get the party coding started …
<form id="register_form" name="register_form" method="post" action="" onSubmit="return createAccount();"> <p> <label for="rq_username" style="text-align:right;">Email Address : </label> <input name="rq_email" type="text" id="rq_email" maxlength="80" class="itext required email" /> </p> <p> <label for="rq_username" style="text-align:right;">Username : </label> <input name="rq_username" type="text" id="rq_username" maxlength="80" class="itext required" /> </p> <p> <label for="rq_password" style="text-align:right;">Password :</label> <input name="rq_password" type="password" id="rq_password" maxlength="80" class="itext required" /> </p> <p> <label for="rq_cpassword" style="text-align:right;">Confirm Password :</label> <input name="rq_cpassword" type="password" id="rq_cpassword" maxlength="80" class="itext required" /> </p> <hr /> <p> <label for="rq_company" style="text-align:right;">Company Name :</label> <input name="rq_company" type="text" id="rq_company" maxlength="80" class="itext required" /> </p> <p> <label for="rq_contact" style="text-align:right;">Contact Person :</label> <input name="rq_contact" type="text" id="rq_contact" maxlength="80" class="itext required" /> </p> <p> <label for="rq_address" style="text-align:right;">Address :</label> <input name="rq_address" type="text" id="rq_address" maxlength="80" class="itext required" /> </p> <p> <label for="rq_address2" style="text-align:right;"> </label> <input name="rq_address2" type="text" id="rq_address2" maxlength="80" class="itext" /> </p> <p> <label for="rq_city" style="text-align:right;">City :</label> <input name="rq_city" type="text" id="rq_city" maxlength="80" class="itext required" /> </p> <p> <label for="rq_state" style="text-align:right;">State :</label> <select name="rq_state" id="rq_state" style="min-width:300px;" class="itext required"> <option value=""></option> <option value="Abia">Abia</option> <option value="Abuja">Abuja</option> <option value="Adamawa">Adamawa</option> <option value="Akwa Ibom">Akwa Ibom</option> <option value="Anambra">Anambra</option> <option value="Bauchi">Bauchi</option> <option value="Bayelsa">Bayelsa</option> <option value="Benue">Benue</option> <option value="Borno">Borno</option> <option value="Cross River">Cross River</option> <option value="Delta">Delta</option> <option value="Ebonyi">Ebonyi</option> <option value="Edo">Edo</option> <option value="Ekiti">Ekiti</option> <option value="Enugu">Enugu</option> <option value="Gombe">Gombe</option> <option value="Imo">Imo</option> <option value="Jigawa">Jigawa</option> <option value="Kaduna">Kaduna</option> <option value="Kano">Kano</option> <option value="Katsina">Katsina</option> <option value="Kebbi">Kebbi</option> <option value="Kogi">Kogi</option> <option value="Kwara">Kwara</option> <option value="Lagos">Lagos</option> <option value="Nasarawa">Nasarawa</option> <option value="Niger">Niger</option> <option value="Ogun">Ogun</option> <option value="Ondo">Ondo</option> <option value="Osun">Osun</option> <option value="Oyo">Oyo</option> <option value="Plateau">Plateau</option> <option value="Rivers">Rivers</option> <option value="Sokoto">Sokoto</option> <option value="Taraba">Taraba</option> <option value="Yobe">Yobe</option> <option value="Zamfara">Zamfara</option> </select> </p> <p> <label for="rq_zip" style="text-align:right;">Postal Code :</label> <input name="rq_zip" type="text" id="rq_zip" maxlength="80" class="itext" /> </p> <p> <label for="rq_landline" style="text-align:right;">Telephone :</label> <input name="rq_landline" type="text" id="rq_landline" maxlength="80" class="itext required" /> </p> <p> <label for="rq_mobile" style="text-align:right;">Mobile Phone :</label> <input name="rq_mobile" type="text" id="rq_mobile" maxlength="80" class="itext" /> </p> <hr /> <div style="margin:5px 0 5px 155px;"> <input type="reset" name="Reset" id="button" value="Clear" /> <input name="rq_register" type="hidden" id="rq_register" value="1" /> <input name="rq_register_btn" type="submit" id="rq_register_btn" value="Create Account" /> </div> </form>
If you have a form like the one above, validating each input sequentially can be so tedious. Below is a good example of sequence validation.
createAccount = function(){ var postForm = document.getElementById('register_form'); if(postForm.rq_email.value == ""){ postForm.rq_email.className = "i_error"; return false; } if(postForm.rq_username.value == ""){ postForm.rq_username.className = "i_error"; return false; } //and so on //and so forth return true; }
I don’t wanna bother about the old method explanation, we are not here to learn about it anyway. Let’s proceed to the use of jQuery for our form validation.
If you are paying good attention, each required field in the form has a class named ‘required‘. This will be use by jQuery whilst validating. The process is easy; instead of doing a sequential validation, we will be using the jQuery .each() method to iterate/loop through each DOM element of the form. Any element that has a class ‘required‘ will go through the validation process; any element that has the class ‘email‘ will go through the email verification process. This example works for empty inputs and email validation respectively; you can create your validation process for other request like valid phone number, valid credit card number and so on.
The script below does the validation:
Firstly, let’s include jQuery on the page:
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4/jquery.min.js"></script>
Then the code:
$(function () { //Remove error class on cursor focus $("#register_form input, #register_form select").focus(function () { $(this).removeClass("i_error"); }); //Disbale submit via enter key for register_form $("#register_form").bind("keypress", function (e) { if (e.keyCode == 13) return false; }); }); //Creat Account createAccount = function () { var submit_it = false; $("#register_form input, #register_form select").each(function (i) { var theParent = $(this).parent().offset(); //Validate empty field if (($(this).val() == '') && $(this).is(".required")) { $(this).addClass("i_error"); $("html,body").animate({ scrollTop: theParent.top - 20 }, 500, 'linear'); submit_it = false; return false; } else { submit_it = true; } //Validate email if ($(this).is(".email")) { prex = $(this).val().indexOf("@"); sux = $(this).val().lastIndexOf("."); if (prex < 1 || sux - prex < 2) { $(this).addClass("i_error"); $("html,body").animate({ scrollTop: theParent.top - 20 }, 500, 'linear'); submit_it = false; return false } } }); if (submit_it === false) { return false; } alert('If you can see this, it means the form is ready to submit to the server, nbut I won\'t submit cause this is just a demo.eeeeehhhhhhhaaaaaa!!!!!'); return false; //Comment this to submit it to the server return true; }
What the script does was:
- iterate through the elements of the form;
- check if the element has the required class;
- then perform validation on the element if the class match the request.
It as simple as that! If the validation goes through, the form will return true by submitting to the server. Click the demo button to see a working example.
You can view the page source to see the complete formatted code.
If you find this helpful, please let me know by leaving a comment.
Thanks for reading.
Awesome! thanks man!